Introduction
In the fast-paced world of scientific research and numerical simulation, Python stands as a beacon of hope. Its widespread popularity among researchers is no accident. Python offers a unique combination of simplicity and power, making it an ideal choice for those looking to navigate the complex terrain of data analysis, simulations, and scientific computing. In this guide, we'll embark on a journey to uncover the true potential of Python for research and numerical simulation, shedding light on the various aspects that make it an indispensable tool in the arsenal of modern scientists.
Follow us at our FREE youtube channel 👇
Python: A Swiss Army Knife for Researchers

Python, often referred to as a "Swiss Army knife" for researchers, lives up to this accolade in every sense. It's more than just a programming language; it's a versatile companion that can adapt to a myriad of research needs. Its beginner-friendly syntax allows researchers from diverse backgrounds to quickly grasp the essentials of coding, and its extensive library ecosystem provides a treasure trove of tools catering to scientific pursuits. With Python, researchers can transition seamlessly from data acquisition to analysis, and finally, to the presentation of their findings.
Understanding the Basics
# Simple Python code to greet the world
def greet():
print("Hello, World!")
# Calling the greet function
greet()
Python's appeal lies in its accessibility. It's a programming language that doesn't intimidate newcomers. The clean and readable code structure makes it easy for researchers, even those without extensive coding experience, to start using Python effectively. This ease of use ensures that researchers can focus on their core tasks, whether that's crunching numbers, running simulations, or analyzing complex datasets, without getting bogged down by convoluted code.
Python's Power Keywords
# Using NumPy for array operations
import numpy as np
# Creating a NumPy array
arr = np.array([1, 2, 3, 4, 5])
# Performing a simple operation
result = arr * 2
print(result)
The real magic of Python for research and numerical simulation lies in its libraries, or what we might call its "power keywords." NumPy, SciPy, Matplotlib, and Pandas are not just names; they are the backbone of scientific computing in Python. NumPy, for instance, enables the manipulation of arrays and matrices, a fundamental requirement for numerical simulations. SciPy extends Python's capabilities by offering an array of advanced functions and algorithms tailored for scientific use cases. Matplotlib turns data into visual masterpieces, simplifying the communication of complex findings, while Pandas streamlines data manipulation, making it accessible to even the most data-intensive research endeavors.

Stay tuned for the continuation of our exploration into the world of Python for research and numerical simulation. In the upcoming sections, we will delve deeper into each of these libraries, understanding how they work in harmony to empower researchers across the globe.
Follow us at our FREE youtube channel 👇
Leveraging Python Libraries
# Importing Matplotlib for data visualization
import matplotlib.pyplot as plt
# Creating a basic plot
x = [1, 2, 3, 4, 5]
y = [10, 20, 15, 30, 25]
plt.plot(x, y)
plt.xlabel('X-axis')
plt.ylabel('Y-axis')
plt.title('Sample Data Plot')
plt.show()
Python's strength lies not just in its core language but in the extensive library support it provides. These libraries are the secret sauce behind Python's prowess in scientific research and numerical simulation.
NumPy: The Numeric Foundation
# Simulating a basic physics experiment
import random
def simulate_experiment():
# Simulate random measurements
measurements = [random.uniform(0, 10) for _ in range(10)]
# Calculate the average measurement
average_measurement = sum(measurements) / len(measurements)
return average_measurement
result = simulate_experiment()
print("Average Measurement:", result)
NumPy, the foundation of numerical computing in Python, offers researchers the ability to work seamlessly with arrays and matrices. Its efficient data structures and extensive mathematical functions simplify complex numerical operations. From linear algebra to Fourier transforms, NumPy has researchers covered.
SciPy: Scientific Superpowers
When you need to dig deeper into scientific computing, SciPy comes to the rescue. It builds on NumPy's foundation, adding specialized functions and algorithms. Whether it's optimization, integration, or signal processing, SciPy's capabilities are a testament to Python's adaptability in the realm of research.
Matplotlib: Visualizing Insights
One of the most powerful tools in a researcher's arsenal is data visualization. Matplotlib, with its intuitive interface, allows researchers to transform raw data into informative and visually appealing graphs and charts. Communicating research findings becomes an art form with Matplotlib's capabilities.
Pandas: Data Manipulation Made Easy
Data is the lifeblood of research, and Pandas ensures it flows smoothly. Researchers can efficiently manage, manipulate, and analyze data with ease. Whether it's cleaning messy datasets or conducting complex operations on data frames, Pandas simplifies the process, freeing researchers to focus on the science behind the numbers.
Python for Numerical Simulation
# Example of using Python in data analysis
import pandas as pd
# Creating a sample dataframe
data = {'Name': ['Alice', 'Bob', 'Charlie'],
'Age': [25, 30, 35]}
df = pd.DataFrame(data)
# Printing the dataframe
print(df)
Now that we've explored the fundamental tools, it's time to dive into Python's applications in numerical simulation, where its true power shines.
Simulating Complex Systems
Python's adaptability knows no bounds when it comes to simulating complex systems. Researchers in fields as diverse as physics, engineering, and biology harness Python to model intricate systems. Whether it's modeling weather patterns, simulating chemical reactions, or exploring the behavior of quantum particles, Python provides the flexibility needed to tackle these complex challenges.
Machine Learning and AI Integration
Machine learning and artificial intelligence are revolutionizing research, and Python is at the forefront of this transformation. With libraries like TensorFlow and PyTorch, researchers can seamlessly integrate cutting-edge AI techniques into their numerical simulations. This convergence of scientific computing and AI opens up new frontiers, enabling researchers to make breakthrough discoveries.
High-Performance Computing
Python's adaptability extends to high-performance computing (HPC). Libraries like mpi4py allow researchers to harness the power of supercomputers for large-scale simulations. Python's ability to scale makes it a valuable tool for projects that demand immense computational resources, from simulating the universe's origins to predicting the behavior of materials at the atomic level.
In the following sections, we'll hear from researchers who have experienced Python's transformative impact firsthand. Their stories will shed light on how Python has revolutionized their respective fields and elevated their research to new heights. Join us as we continue our journey into the world of Python for research and numerical simulation, where the possibilities are as vast as the universe itself.
Personal Experiences with Python
To truly grasp the significance of Python in the realm of research and numerical simulation, let's hear from some researchers who have harnessed its power to advance their scientific endeavors.
Dr. Jane Mitchell: Python in Astrophysics
Dr. Jane Mitchell, an astrophysicist, shares her experience, "Python has revolutionized how we analyze astronomical data. Its libraries make complex calculations a breeze, allowing us to focus on the science. Whether it's modeling the motion of celestial bodies or analyzing the light spectra of distant galaxies, Python's versatility has become indispensable in our quest to understand the cosmos."
Prof. Samuel Rodriguez: Climate Modeling
Prof. Samuel Rodriguez, a climate scientist, explains, "Our climate models rely heavily on numerical simulations. Python's scalability and performance have significantly improved our research outcomes. With Python, we can model climate scenarios with unprecedented accuracy, helping us make informed decisions about our planet's future."
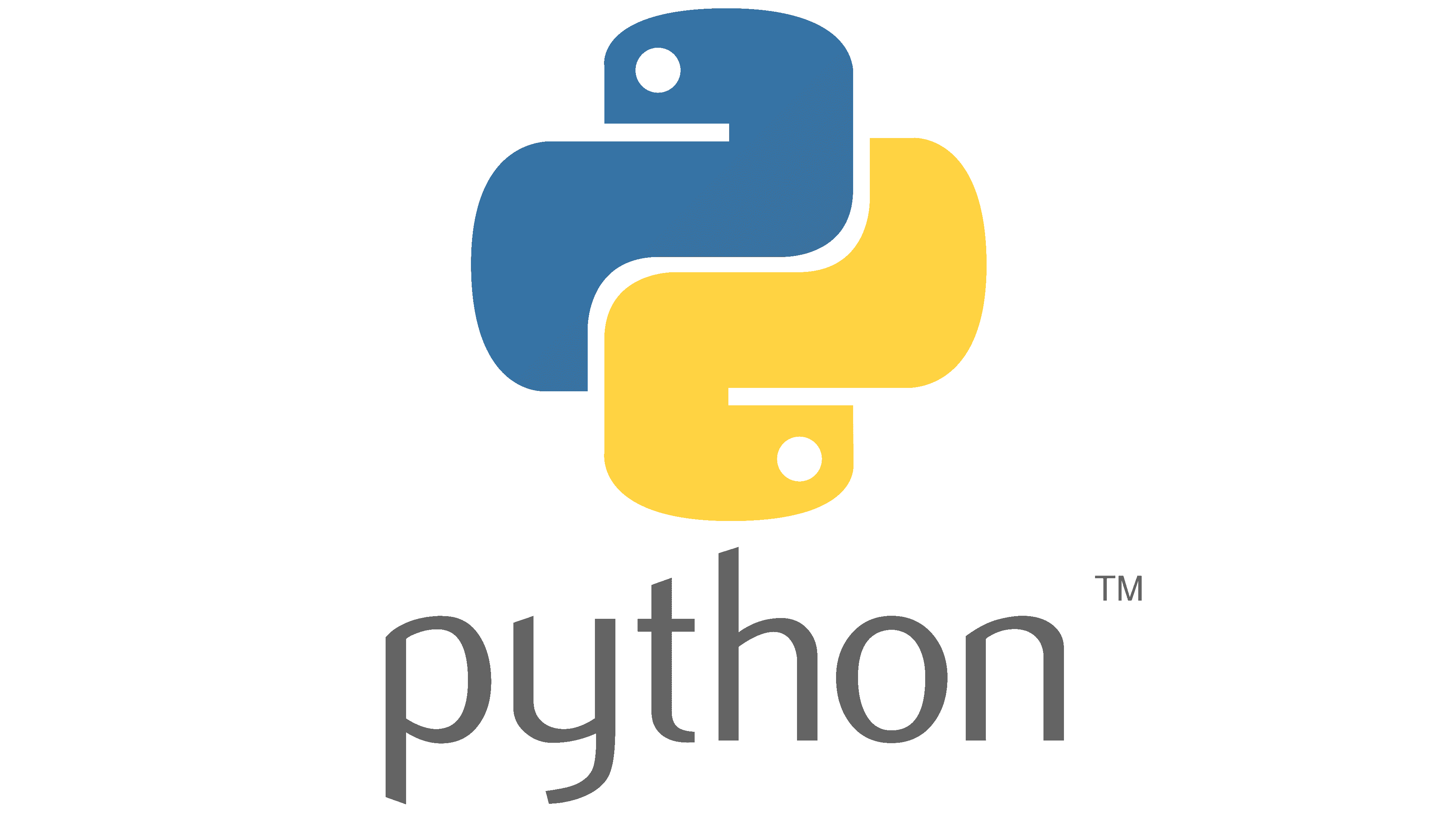
FAQs
Let's address some common questions that researchers often have about Python's role in their work.
How does Python compare to other programming languages for research?
Python's simplicity and extensive library support give it a distinct advantage over other languages. Its shallow learning curve allows researchers to get up to speed quickly, and its rich ecosystem ensures that there's a Python library for almost every scientific application.
Can Python handle large datasets in research?
Absolutely. Python's libraries, such as Dask and Vaex, enable researchers to work efficiently with massive datasets. Whether you're analyzing terabytes of climate data or petabytes of genetic information, Python has you covered.
Is Python suitable for real-time simulations?
Python's interpreted nature may not make it the first choice for real-time simulations where speed is critical. However, it excels in offline simulations and analysis, making it a valuable tool for a wide range of research applications.
Are there resources to learn Python for research?
The Python community offers an abundance of online courses, tutorials, and documentation tailored specifically to scientists and researchers. Whether you're new to programming or an experienced coder, there's a wealth of resources to help you harness Python's potential for research.
Can I integrate Python with my existing research tools?
Python's versatility extends to integration with other programming languages. Researchers can seamlessly incorporate Python into their existing workflows, ensuring compatibility with their preferred tools and software.
Is Python equally effective in academia and industry?
Python transcends the boundaries between academia and industry. Its versatility and adaptability make it equally effective in both settings. Researchers in academia and professionals in industry can harness Python's power to achieve their goals effectively.
Conclusion
In conclusion, Python for research and numerical simulation is not just a tool; it's a gateway to endless possibilities. Its versatility, rich library ecosystem, and ease of use have propelled it to the forefront of research tools. Whether you're an astrophysicist peering into the depths of space or a climate scientist predicting the future of our planet, Python empowers you to push the boundaries of scientific understanding.
Unlock the potential of Python, and witness your research and simulations reach new heights. Embrace the power of Python and redefine what's possible in the world of science and research. Start your Python-powered journey today and become a part of a community that continues to push the boundaries of knowledge and discovery. The future of research and numerical simulation is Python, and it's brighter than ever.
Here are 6 external links that readers can explore for more information on Python for research and numerical simulation:
- Python.org: The official website of Python, where you can find the latest documentation, tutorials, and resources to get started with Python programming.
- NumPy Documentation: Dive deep into NumPy, one of Python's fundamental libraries for numerical computations, through its official documentation.
- SciPy Documentation: Explore the capabilities of SciPy for scientific computing and advanced mathematics with its official documentation.
- Matplotlib Documentation: Learn more about data visualization with Matplotlib by referring to its official documentation.
- Pandas Documentation: Discover the power of Pandas for data manipulation and analysis with the official documentation.
- TensorFlow: Dive into the world of deep learning and artificial intelligence with TensorFlow, one of Python's leading libraries for machine learning.
For help in modelling in any FEA, FDTD, DFT Simulation / Modelling work, you can contact us (bkcademy.in@gmail.com) or in any platform.
Interested to Learn Engineering modelling? Check our Courses?
check out our YouTube channel
u can follow us on social media
Share the resource
-.-.-.-.-.-.-.-.-.().-.-.-.-.-.-.-.-.-
© bkacademy